Loading... https://leetcode.cn/problems/binary-tree-paths/description/ ## 题目 给你一个二叉树的根节点 `root` ,按 **任意顺序** ,返回所有从根节点到叶子节点的路径。 **叶子节点** 是指没有子节点的节点。 **示例 1:** 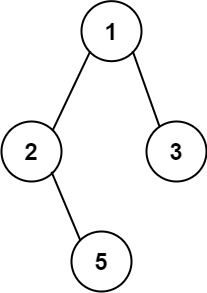 **输入:** root = [1, 2, 3, null, 5] **输出:**["1->2->5","1->3"] **示例 2:** **输入:** root = [1] **输出:**["1"] **提示:** - 树中节点的数目在范围 `[1, 100]` 内 - `-100 <= Node.val <= 100` ## 思路 采用深度优先遍历法,从根节点开始,将当前节点已经遍历到的所有节点路径传递给子节点,直到最后的子节点为叶子节点时将整条路径添加到结果中。 ## 代码 ```java /** * Definition for a binary tree node. * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode() {} * TreeNode(int val) { this.val = val; } * TreeNode(int val, TreeNode left, TreeNode right) { * this.val = val; * this.left = left; * this.right = right; * } * } */ class Solution { public List<String> binaryTreePaths(TreeNode root) { List<String> paths = new ArrayList<>(); constructPaths(root, "", paths); return paths; } public void constructPaths(TreeNode root, String path, List<String> paths) { if (root != null) { path = path + Integer.toString(root.val); if (root.left != null || root.right != null) { path = path + "->"; constructPaths(root.left, path, paths); constructPaths(root.right, path, paths); } else { paths.add(path); } } } } ``` 最后修改:2023 年 01 月 21 日 © 允许规范转载 打赏 赞赏作者 支付宝微信 赞 如果觉得我的文章对你有用,请我喝杯咖啡吧。